Project: spi-flash-programmer Author: nfd File: spiflashprogrammerclient.py License: Creative.
Download the Serial Example: DSIPythonEX (zipped .py)

After python and the pyserial module has been installed on your system, this example code will send connect, send, and receive commands from our products:
# for PYTHON 3+ with pySerial module installed# DS INSTRUMENTS 2017 PYTHON SCPI REMOTE CONTROL EXAMPLE
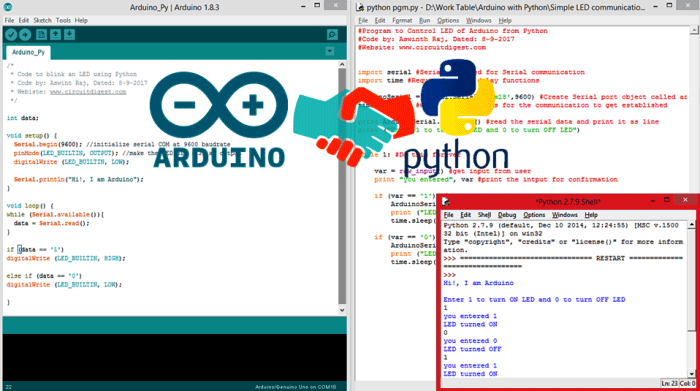
import serial # use the serial module (https://pypi.python.org/pypi/pyserial)
import time # delay functions
badCommandResponse = b'[BADCOMMAND]rn’ # response if a command failed (b makes it into bytes)
- Install Pyserial. Before installing Pyserial, we need to get pip: sudo apt install python-pip. Now we can go on to install Pyserial: python -m pip install pyserial Test installation and read console. To read our console, we need to connect the Raspberry Pi USB port to Arduino one. For testing purposes, I'll show you results from my weather system.
- Python Serial Communication (pyserial) Python Language Tutorial Python Language Pedia Tutorial. Data = ser.readline to read the data from serial device while something is being written over it. #for python2.7 data = ser.read(ser.inWaiting) #for python3 ser.read(ser.inWaiting).
ser = serial.Serial(“COM79”, 115200, timeout=1) #Change the COM PORT NUMBER to match your device
if ser.isOpen(): # make sure port is open
print(ser.name + ‘ open…’) # tell the user we are starting
ser.write(b’*IDN?n’) # send the standard SCPI identify command
myResponse = ser.readline() # read the response
print(b’Device Info: ‘ + myResponse) # print the unit information
time.sleep(0.1) # delay 100ms
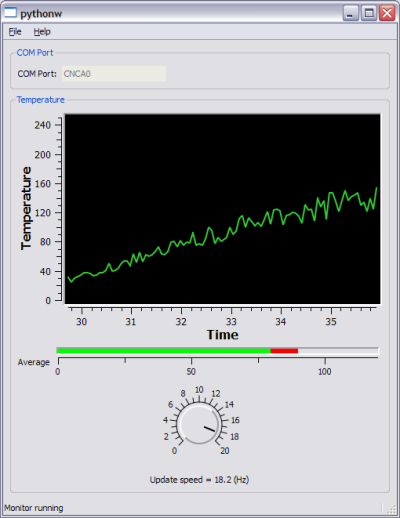
ser.write(b’PHASE?n’) # try asking for phase
myResponse = ser.readline() # gather the response
if myResponse != badCommandResponse: #is this is not a phase shifter why print the error
print(b’Phase=’ +myResponse)
time.sleep(0.1) # delay 100ms
ser.write(b’FREQ:CW?n’) # try asking for signal generator setting
myResponse = ser.readline() # gather the response
if myResponse != badCommandResponse: # is this is not a signal generator why print the error
print(b’Frequency=’ + myResponse)
time.sleep(0.1) # delay 100ms
ser.write(b’ATT?n’) # try asking for step attenuator setting
myResponse = ser.readline() # gather the response
if myResponse != badCommandResponse: # is this is not an attenuator why print the error
Python Pyserial Read Example
print(b’Attenuation=’ + myResponse)
time.sleep(0.1) # delay 100ms
Python Pyserial Readline Example Python
ser.write(b’FREQ:CW 3GHZn’) #lets change a setting now!